Understanding the Differences Between Arrays and Vectors in Rust
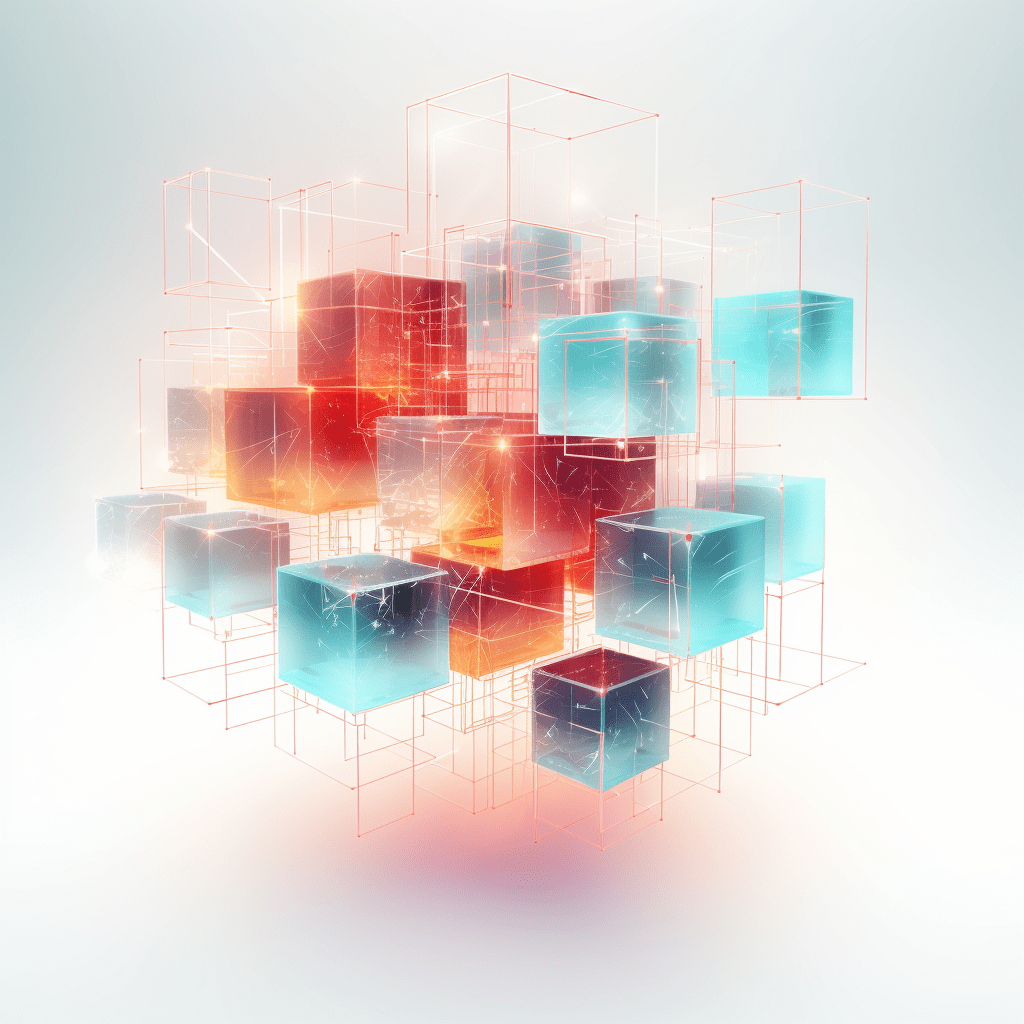
Rust is a systems programming language that is designed to be safe, fast, and concurrent. One of the fundamental concepts in Rust is the use of collections to store data. Two of the most common collection types in Rust are arrays and vectors. While both arrays and vectors serve a similar purpose, there are some key differences between the two that developers need to understand in order to use them effectively.
In this article, we'll explore the differences between arrays and vectors in Rust. We'll take a look at their properties, use cases, and performance characteristics to help you make informed decisions when choosing between the two.
Arrays and vectors are both data structures that allow developers to store a collection of elements of the same type. However, they differ in several ways.
Arrays in Rust are fixed-size collections of elements that are allocated on the stack. The size of an array is determined at compile time and cannot be changed at runtime. Arrays can be accessed using an index, starting from 0. The syntax for defining an array in Rust is as follows:
let my_array: [i32; 3] = [1, 2, 3];
In this example, my_array
is an array of 3 integers. The i32
indicates that the array holds 32-bit signed integers, and 3
indicates that the array has a length of 3.
Arrays are useful when you know the exact number of elements that you need to store and that number won't change. They are also useful when you need to allocate memory on the stack, which can be faster than allocating memory on the heap.
Vectors, on the other hand, are dynamic-size collections of elements that are allocated on the heap. Vectors can grow or shrink in size at runtime as elements are added or removed. Vectors can be accessed using an index, like arrays, or using methods like push
and pop
. The syntax for defining a vector in Rust is as follows:
let mut my_vector: Vec<i32> = Vec::new();
my_vector.push(1);
my_vector.push(2);
my_vector.push(3);
In this example, my_vector
is an empty vector of integers. The mut
keyword indicates that the vector is mutable, and Vec<i32>
specifies the type of elements that the vector can hold. The push
method is then used to add elements to the vector.
Vectors are useful when you don't know the exact number of elements that you need to store or when that number may change at runtime. They are also useful when you need to allocate memory on the heap, which can be more flexible than allocating memory on the stack.
Another important difference between arrays and vectors is their performance characteristics. Since arrays are allocated on the stack, they can be faster to access and manipulate than vectors, which are allocated on the heap. However, arrays are limited in size, and if you need to store a large number of elements, a vector may be a better choice despite the performance hit.
It's also worth noting that Rust provides a range of other collection types, such as slices, hash maps, and linked lists, that can be used in different scenarios depending on the requirements of your program.
In summary, arrays and vectors are both useful collection types in Rust, but they have different properties and use cases. Arrays are fixed-size collections that are allocated on the stack and are useful when you know the exact number of elements that you need to store. Vectors, on the other hand, are dynamic-size collections that are allocated on the heap and are useful when you don't know the exact number of elements that you need to store or when that number may change at runtime.
When deciding between arrays and vectors, it's important to consider factors such as performance, memory usage, and flexibility. Rust provides a range of other collection types, such as slices, hash maps, and linked lists, that can be used in different scenarios depending on the requirements of your program.
By understanding the differences between arrays and vectors, and other collection types in Rust, you can make informed decisions when choosing the appropriate data structure for their program.
Member discussion